scikits.learn: machine learning in python¶
Easy-to-use and general-purpose machine learning in Python
scikits.learn is a Python module integrating classic machine learning algorithms in the tightly-knit world of scientific Python packages (numpy, scipy, matplotlib).
It aims to provide simple and efficient solutions to learning problems that are accessible to everybody and reusable in various contexts: machine-learning as a versatile tool for science and engineering.
Features: |
|
---|---|
License: | Open source, commercially usable: BSD license (3 clause) |
A simple Example: recognizing hand-written digits
import pylab as pl
from scikits.learn import datasets, svm
digits = datasets.load_digits()
for index, (image, label) in enumerate(zip(digits.images, digits.target)[:4]):
pl.subplot(2, 4, index+1)
pl.imshow(image, cmap=pl.cm.gray_r)
pl.title('Training: %i' % label)
n_samples = len(digits.images)
data = digits.images.reshape((n_samples, -1))
classifier = svm.SVC()
classifier.fit(data[:n_samples/2], digits.target[:n_samples/2])
for index, image in enumerate(digits.images[n_samples/2:n_samples/2+4]):
pl.subplot(2, 4, index+5)
pl.imshow(image, cmap=pl.cm.gray_r)
pl.title('Prediction: %i' % classifier.predict(image.ravel()))
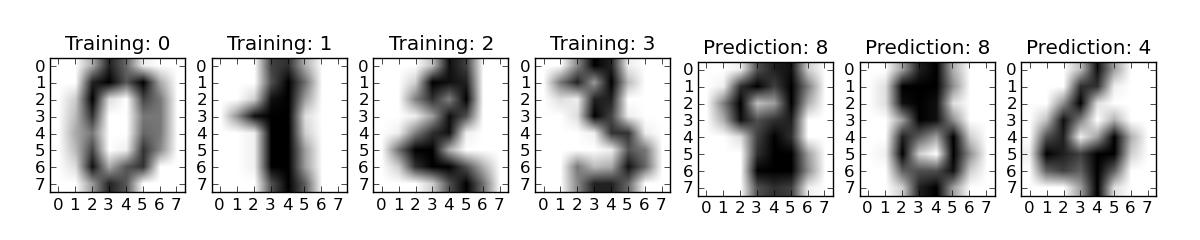
Warning
This documentation is relative to the development version, documentation for the stable version can be found here